Introduction
Organizations face a critical challenge when using AI: balancing the need for powerful AI capabilities with stringent data privacy requirements. As AI models become more sophisticated, the complexity of managing development environments while ensuring data security has become a significant hurdle for many teams. AI engineers and data scientists want to work with the latest cutting-edge models, whether they be open source or proprietary LLMs, but often find themselves spending valuable time configuring environments and wrestling with dependency conflicts, all while trying to maintain the security standards their organizations demand.
Enter HP AI Studio and it’s 1-click containerization, a solution that transforms how developers work with sophisticated AI models like the recently released DeepSeek models in a secure, local environment. This innovation directly addresses the dual challenges of development environment management and data privacy, allowing teams to focus on what matters most – building exceptional AI applications.
In this article, you'll learn how to leverage HP AI Studio's containerization capabilities to create reproducible, secure development environments for your AI projects in seconds, not hours. We'll walk through the entire process, from initial setup to advanced implementation, showing you how to save valuable development time while maintaining the highest standards of data privacy.
Technical Background
The Environment Challenge
The modern AI development stack is inherently complex, requiring careful orchestration of multiple dependencies, libraries, and model weights. Traditional approaches to environment management, such as virtual environments, often fall short when dealing with the specific requirements of large language models like the newly released DeepSeek series of models. Developers frequently encounter version conflicts, incompatible dependencies, and inconsistent behavior across different machines.
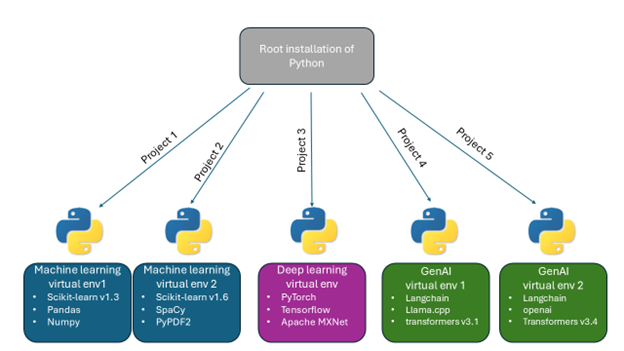
Current Limitations
- Traditional approaches to managing AI development environments typically involve:
- Manual virtual environment creation and management
- Complex dependency resolution processes
- Inconsistent environment reproduction across team members
- Time-consuming setup procedures for each new project
- Limited isolation between the host system and AI development environment
These limitations not only slow down development but also create security vulnerabilities when working with sensitive data and powerful AI models, potentially exposing local host machine file systems and corporate network processes.
Setting Up Your Containerized Environment in HP AI Studio
HP AI Studio simplifies AI developer workflows by moving beyond virtual environments and creating containerized development environments. Normally this would involve an equally cumbersome Docker setup, but with HP AI Studio we’ve condensed this down into a single click.
Downloading DeepSeek to my local machine
To begin using DeepSeek within an HP AI Studio containerized environment go to HuggingFace and select the specific model repo you’d like to use. For the purposes of this demonstration I used the DeepSeek-R1-Distill-Qwen-1.5B (deepseek-ai/DeepSeek-R1-Distill-Qwen-1.5B · Hugging Face). Save the model files somewhere on your local machine where you can access them later. I’ve also included code (See implementation below) that will allow you to download during the implementation phase of the project.
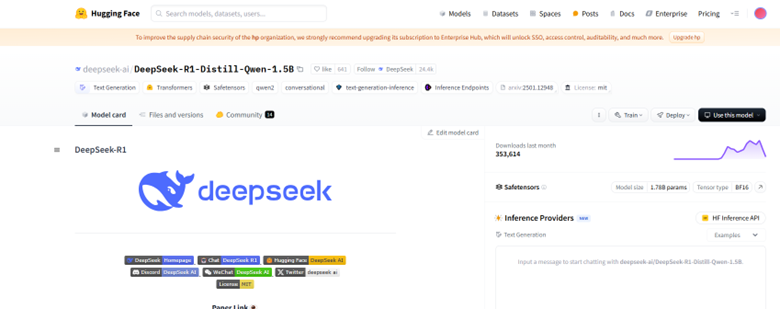
Basic Setup Process in HP AI Studio
- Selecting a pre-configured workspace
With HP AI Studio we provide a number of pre-configured development images that contain all the tools you need for your different AI workflows. Once you’ve selected one of these, you are ready to build your containerized development environment. I’ve selected the ‘Local GenAI’ image because it provides me common GenAI libraries, e.g., Langchain, llama.cpp, and local vector database packages.
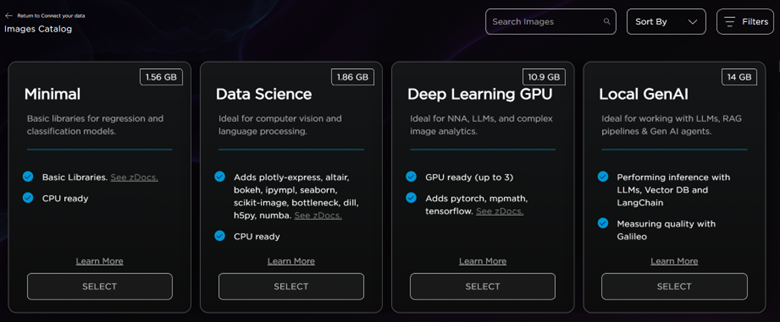
- Getting DeepSeek model files into HP AI Studio
From here, I can simply point to the location on my local machine where I downloaded and saved the DeepSeek model and configuration files from HuggingFace. You don’t point to specific files, just the local directory itself in the ‘Local Folder’ section.
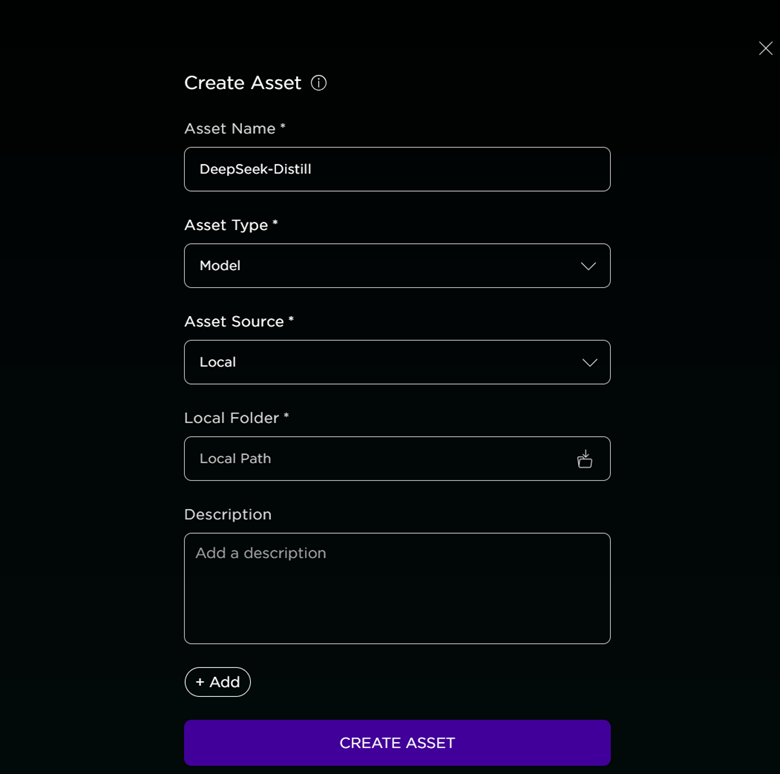
- Container Configuration
Next comes the all-important containerization step which will isolate my local development environment on my machine.
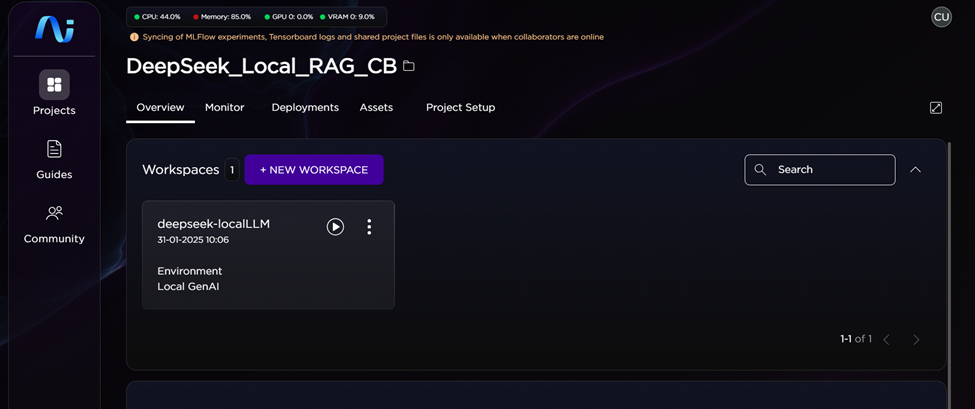
By simply pressing the ‘Play’ button highlighted above AI Studio builds the container for you.
The container automatically includes:
- All necessary Python dependencies
- Optimized environment variables
- Security configurations to ensure that processes and model execution taking place inside the container does not impact anything on a local host machine
Practical Example: Building a secure AI chatbot
Let's walk through building a privacy-preserving RAG based application using DeepSeek in our containerized environment.
As helpful context, I work as a product manager at HP so I wanted to set up a demonstration that resonated with me, but you could replace the dataset I’ve used within any dataset that it useful for you. The dataset I’m using is a from blog post around different growth tactics a product manager might use to help grow the core business for a software product.
Here you’ll find the code I used to build the RAG based system, as well as some AI evaluations of my system using Galileo, an HP partner and industry leader in AI evaluation and observability (What is Galileo? - Galileo)
Implementation Steps
### Load and install any additional libraries needed
import os
!pip install PyPDF2
!pip install faiss-gpu
!pip install langchain_communitylKH1] sCB2]
from urllib.request import urlretrieve
import numpy as np
from langchain_community.embeddings import HuggingFaceBgeEmbeddings
from langchain_community.llms import HuggingFacePipeline
from langchain_community.document_loaders import PyPDFLoader
from langchain_community.document_loaders import PyPDFDirectoryLoader
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain_community.vectorstores import FAISS
from langchain.chains import RetrievalQA
from langchain.prompts import PromptTemplate
import PyPDF2
import faiss
from huggingface_hub import snapshot_download
###Download the DeepSeek and embedding models locally to AI Studio
local_model_dir = "/datafabric/DeepSeek-Distill-Qwen1.5B”
local_embed_dir = "/home/jovyan/datafabric/mxai-embed",
repo_id = 'deepseek-ai/DeepSeek-R1-Distill-Qwen-1.5B' #DeepSeek model
embed_repo_id = ‘mixedbread-ai/mxbai-embed-large-v1’ #embedding model
snapshot_download(repo_id=repo_id, local_dir=local_model_dir,repo_type="model")
snapshot_download(repo_id =embed_repo_id, local_dir=local_embed_dir,repo_type=”model”)
### Bring in a PDF data source and set up code to collate pages if PDF extends across multiple pages
pdfFileObject = open("growth_loops_product_school.pdf", 'rb')
pdfReader = PyPDF2.PdfReader(pdfFileObject)
count = pdfReader.pages
full_doc = ''
for i in range(len(count)):
page = pdfReader.pages i]
full_doc += page.extract_text()
### Previous output 'full doc' is just one big string and a 'Document' object
### is required for some later langchain calls. So, define a function that creates
### the Document object
def generate_tokens(s):
text_splitter = RecursiveCharacterTextSplitter(chunk_size=500, chunk_overlap=50)
splits = text_splitter.split_text(s)
return text_splitter.create_documents(splits)
### Create document chunks
docs_after_split = generate_tokens(full_doc)
### Encode the document chunks using an embedding model I also brought into HP AI Studio
huggingface_embeddings = HuggingFaceBgeEmbeddings(
model_name="/home/jovyan/datafabric/mxai-embed",
model_kwargs={'device':'gpu'},
encode_kwargs={'normalize_embeddings': True},
)
### Set up local vector store and retriever
vectorstore = FAISS.from_documents(docs_after_split, huggingface_embeddings)
retriever = vectorstore.as_retriever(search_type="similarity", search_kwargs={"k": 4})
### Set up DeepSeek model pipeline
from transformers import pipeline
pipe = pipeline(task = "text-generation",
model='/home/jovyan/datafabric/DeepSeek-Distill-Qwen1.5B',
temperature = 0.15,
max_new_tokens = 300,
device = -1)
from langchain_community.llms.huggingface_pipeline import HuggingFacePipeline
hf = HuggingFacePipeline(pipeline=pipe)
llm = hf
### Set up a prompt template which will be used with the LLM
from langchain.prompts import ChatPromptTemplate
from langchain.schema import StrOutputParser
from langchain.schema.runnable import RunnablePassthrough
from typing import List
from langchain.schema.document import Document
def format_docs(docs: ListnDocument]) -> str:
return "\n\n".join(
prompt_template = """
{context}
Question: {question}
Helpful Answer:
"""
PROMPT = ChatPromptTemplate.from_template(prompt_template)
chain = {"context": retriever | format_docs, "question": RunnablePassthrough()} | PROMPT | llm | StrOutputParser()
### Set up model evaluation using Galileo (HP Partner for AI Evals)
import promptquality as pq
os.environp'GALILEO_API_KEY'] = "your api Key"
galileo_url = "https://www.galileo.io/"
pq.login(galileo_url)
Scorers = Lpq.Scorers.context_adherence_luna,
pq.Scorers.correctness,
pq.Scorers.pii,
pq.Scorers.completeness_luna,
pq.Scorers.chunk_attribution_utilization_luna]
prompt_handler = pq.GalileoPromptCallback(
project_name="growth_RAG",
scorers=Scorers
)
### Run your chain experiments across multiple inputs with the galileo callback
inputs = r
"Why are growth loops important?",
"What kind of growth loops does Dropbox use?",
"What are some limitations of the AARRR framework?",
"What are network effects?"
chain.batch(inputs, config=dict(callbacks=dprompt_handler]))
### Publish the results of your run to Galileo
prompt_handler.finish()```
Expected Outputs
I won’t go over all the expected outputs but using the last question I provide the LLM ‘What are network effects?’, I would expect a basic explanation of what network effects are in the context of growing a software product and why they are important.
Using DeepSeek I was able to get exactly that from the dataset I provided it.
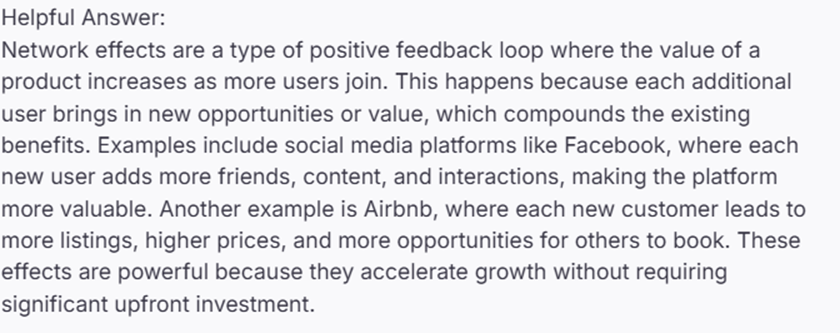
While I need to potentially test model performance further, I feel confident that using DeepSeek within HP AI Studio I have built a basic RAG based system that provides answers I can be confident in, all while protecting my local machine from any potential security concerns of using this cutting-edge open source LLM.
Conclusion and Additional Considerations
HP AI Studio's 1-click containerization revolutionizes how teams work with sophisticated AI models like DeepSeek. By eliminating the complexity of environment setup and management, teams can save significant time while maintaining robust security standards. The ability to create consistent, isolated development environments with a single click not only accelerates development but also ensures that privacy and security remain at the forefront of AI implementation.
Constainers vs. Virtual Environment
Containers offer several key advantages over virtual environments:
- Complete isolation: Full system-level isolation ensures better security
- Reproducibility: Guaranteed consistent environment across all deployments
- Resource efficiency: Better resource management than virtual machines
- Version control: Easier to track and manage environment changes
- Scalability: Seamless scaling from development to production
Ready to transform your own AI development workflow?
Contact HP's AI Solutions team to learn more about how HP AI Studio can help your organization build secure, efficient AI applications. You can find contact information here Z by HP AI Studio | HP Official Site to schedule a demonstration.
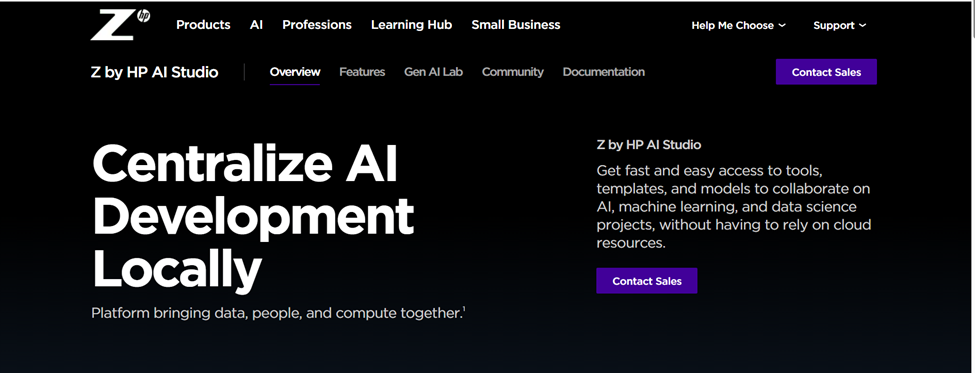